Python has become one of the most popular programming languages in recent years, thanks to its simplicity, versatility, and growing ecosystem of libraries and tools. As a Python developer, setting up a robust and efficient development environment is crucial for productivity and success. In this comprehensive blog post, we’ll guide you through the process of setting up a Python development environment, covering everything from choosing an IDE to configuring version control.
Overview of Python Development
Python is a high-level, interpreted programming language that is widely used for a variety of applications, including web development, data analysis, machine learning, and automation. One of the key advantages of Python is its ease of use and readability, which makes it an excellent choice for beginners and experienced developers alike.
Advantages of Python
- Simplicity: Python has a clean and readable syntax, making it easy to learn and understand.
- Versatility: Python can be used for a wide range of applications, from web development to scientific computing.
- Large Ecosystem: Python has a vast and growing ecosystem of libraries and tools, which can be used to extend its functionality.
- Cross-Platform: Python is a cross-platform language, meaning it can be run on a variety of operating systems, including Windows, macOS, and Linux.
Common Use Cases for Python
- Web Development: Python is a popular choice for web development, thanks to the popularity of frameworks like Django and Flask.
- Data Analysis and Visualization: Python’s data analysis and visualization libraries, such as NumPy, Pandas, and Matplotlib, make it a powerful tool for data-driven applications.
- Machine Learning and Artificial Intelligence: Python’s robust machine learning and AI libraries, such as TensorFlow and Scikit-learn, have made it a go-to language for these fields.
- Automation and Scripting: Python’s simplicity and versatility make it an excellent choice for automating repetitive tasks and writing scripts.
Choosing an IDE
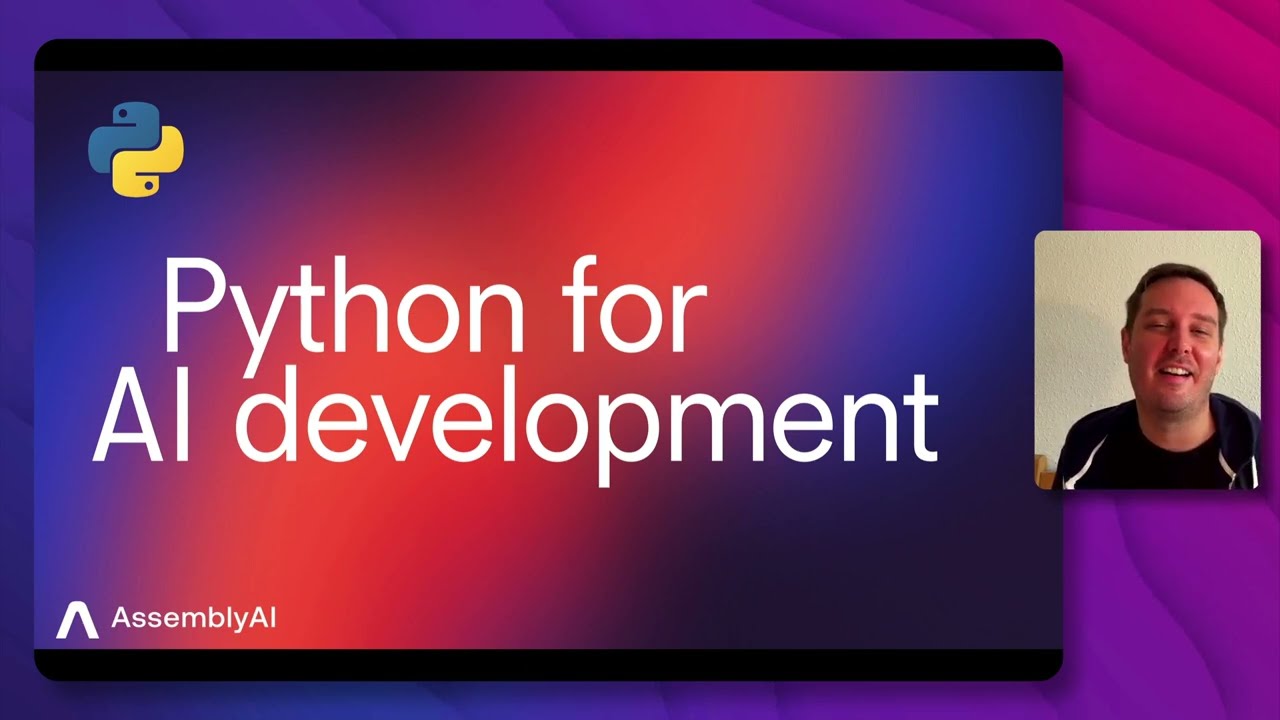
An Integrated Development Environment (IDE) is a software application that provides a comprehensive set of tools and features for writing, testing, and debugging code. When it comes to Python development, there are several popular IDEs to choose from, each with its own strengths and features.
Popular Python IDEs
- PyCharm: PyCharm is a powerful IDE developed by JetBrains, which offers a range of features for Python development, including code assistance, debugging tools, and support for popular Python frameworks.
- Visual Studio Code: Visual Studio Code is a free, open-source IDE developed by Microsoft that has become increasingly popular for Python development. It offers a wide range of extensions and customization options.
- Spyder: Spyder is a free, open-source IDE that is designed specifically for scientific and data-driven Python development. It includes features like a variable explorer, a code editor, and built-in support for popular data analysis libraries.
- Jupyter Notebook: Jupyter Notebook is a web-based interactive computing environment that allows you to create and share documents that contain live code, visualizations, and narrative text. It’s particularly useful for data analysis and exploration.
- Sublime Text: Sublime Text is a popular code editor that can be used for Python development. While it doesn’t have all the features of a full-fledged IDE, it is lightweight, customizable, and offers a range of plugins and extensions.
Factors to Consider when Choosing an IDE
When choosing an IDE for Python development, there are several factors to consider:
- Functionality: What features and tools does the IDE offer, and how well do they meet your needs?
- Ease of Use: How intuitive and user-friendly is the IDE’s interface?
- Performance: How responsive and efficient is the IDE, especially when working with large projects or datasets?
- Customization: Can you customize the IDE’s appearance, keybindings, and other settings to suit your preferences?
- Integration: Does the IDE integrate well with other tools and services you use, such as version control systems and cloud-based platforms?
Installing Python

Before you can start developing in Python, you’ll need to install the Python interpreter on your computer. The Python interpreter is the software that executes Python code and provides access to the Python standard library and third-party packages.
Downloading Python
The latest version of Python can be downloaded from the official Python website (https://www.python.org/downloads/). The website provides installers for Windows, macOS, and various Linux distributions.
Installing Python on Windows
- Download the Installer: Visit the Python website and download the latest version of Python for Windows.
- Run the Installer: Double-click the downloaded installer and follow the on-screen instructions to install Python. Make sure to select the “Add Python to PATH” option during the installation process.
- Verify the Installation: Open the Windows Command Prompt and type
python --version
. If the installation was successful, you should see the version of Python that you installed.
Installing Python on macOS
- Download the Installer: Visit the Python website and download the latest version of Python for macOS.
- Run the Installer: Double-click the downloaded installer and follow the on-screen instructions to install Python.
- Verify the Installation: Open the Terminal application and type
python3 --version
. If the installation was successful, you should see the version of Python that you installed.
Installing Python on Linux
The process for installing Python on Linux varies depending on the distribution, but generally, you can use the system’s package manager to install Python. For example, on Ubuntu, you can use the following command:
sudo apt-get install python3
After the installation, you can verify the installation by typing python3 --version
in the terminal.
Setting up Virtual Environments
When working on multiple Python projects, it’s important to manage your dependencies and avoid conflicts between different versions of packages or libraries. Virtual environments are a powerful tool for accomplishing this task.
What are Virtual Environments?
Virtual environments are self-contained Python environments that allow you to install packages and dependencies specific to a project, without affecting other projects or the system-wide Python installation.
Benefits of Using Virtual Environments
- Dependency Management: Virtual environments allow you to install and manage packages independently for each project, preventing conflicts between different versions of the same package.
- Reproducibility: Virtual environments ensure that your development environment is consistent across different machines, making it easier to share and collaborate on projects.
- Isolation: Virtual environments isolate your project’s Python installation and dependencies, preventing them from interfering with other projects or the system-wide Python installation.
Creating and Managing Virtual Environments
There are several tools available for creating and managing virtual environments, such as venv
(built-in to Python 3) and conda
(part of the Anaconda distribution).
Using venv
- Create a Virtual Environment: Open a terminal or command prompt and run the following command to create a new virtual environment:
python -m venv my_env
This will create a new directory calledmy_env
containing the virtual environment.
- Activate the Virtual Environment: To activate the virtual environment, run the following command:
- On Windows:
my_env\Scripts\activate
- On macOS/Linux:
source my_env/bin/activate
- On Windows:
- Install Packages: Once the virtual environment is activated, you can install packages using
pip
as you normally would.
Using conda
If you’re using the Anaconda distribution of Python, you can use the conda
command to create and manage virtual environments:
- Create a Virtual Environment: Open a terminal or command prompt and run the following command to create a new virtual environment:
conda create -n my_env python=3.9
This will create a new virtual environment namedmy_env
with Python 3.9 installed.
- Activate the Virtual Environment: To activate the virtual environment, run the following command:
conda activate my_env
- Install Packages: Once the virtual environment is activated, you can install packages using
conda
orpip
as you normally would.
Installing Packages and Libraries
Python’s rich ecosystem of packages and libraries is one of the key reasons for its popularity. These packages provide a wide range of functionality, from data analysis and machine learning to web development and scientific computing.
Using pip
pip
is the package installer for Python, and it is the primary tool for installing and managing Python packages. To install a package using pip
, simply open a terminal or command prompt (with your virtual environment activated) and run the following command:
pip install
For example, to install the popular numpy
library, you would run:
pip install numpy
Handling Package Dependencies
Many Python packages have their own dependencies on other packages or libraries. When you install a package, pip
will automatically install the required dependencies as well. However, it’s important to keep track of your project’s dependencies to ensure reproducibility and avoid version conflicts.
Saving and Restoring Dependencies
To save the current state of your project’s dependencies, you can use the pip freeze
command to generate a requirements file:
pip freeze > requirements.txt
This will create a requirements.txt
file containing a list of all the installed packages and their versions.
To restore the dependencies from the requirements.txt
file, you can use the following command:
pip install -r requirements.txt
This will install all the packages listed in the requirements.txt
file, ensuring that your development environment is consistent across different machines.
Configuring Version Control
Version control is an essential tool for any software development project, including Python development. It allows you to track changes to your code, collaborate with other developers, and manage the project’s history.
Git and GitHub
Git is the most widely used version control system, and GitHub is a popular hosting service for Git repositories. If you’re new to Git and GitHub, you can start by creating a free account on GitHub and familiarizing yourself with the basic Git commands.
Initializing a Git Repository
To initialize a Git repository for your Python project, follow these steps:
- Open a terminal or command prompt and navigate to your project’s directory.
- Run the following command to initialize a new Git repository:
git init
- Add your project files to the repository using the
git add
command:
git add .
- Commit your initial changes with a descriptive message:
git commit -m "Initial commit"
Connecting to a Remote Repository
Once you have a local Git repository set up, you can connect it to a remote repository on a service like GitHub:
- Create a new repository on GitHub.
- Copy the repository’s URL (e.g.,
https://github.com/username/my-project.git
). - In your terminal, run the following command to link your local repository to the remote one:
git remote add origin https://github.com/username/my-project.git
- Push your local commits to the remote repository:
git push -u origin master
Branching and Collaboration
Git’s branching feature allows you to work on different features or bug fixes simultaneously, without affecting the main codebase. When working with a team, you can create a new branch for each task or feature, and then merge the changes back into the main branch (usually master
or main
) when the work is complete.
To create a new branch and switch to it, run the following command:
git checkout -b my-feature
You can then make your changes, commit them, and push the branch to the remote repository:
git push origin my-feature
Tips for Efficient Python Development
As you set up your Python development environment, here are some tips to help you work more efficiently and productively:
Keyboard Shortcuts and Customization
- Learn and use keyboard shortcuts for common tasks in your IDE, such as code formatting, file navigation, and debugging.
- Customize your IDE’s settings and keybindings to match your preferences and workflow.
Debugging and Error Handling
- Become familiar with your IDE’s debugging tools, such as breakpoints, step-through execution, and variable inspection.
- Implement robust error handling in your code, using techniques like try-except blocks and custom exception classes.
Automated Testing
- Write unit tests for your code using frameworks like unittest or pytest to catch bugs early and ensure code reliability.
- Set up continuous integration (CI) tools, such as GitHub Actions or Travis CI, to automatically run your tests whenever you push changes to your repository.
Code Formatting and Linting
- Use a code formatter like black or autopep8 to maintain a consistent code style across your project.
- Integrate a linter like pylint or flake8 into your development workflow to catch common coding errors and style issues.
Continuous Learning
- Stay up-to-date with the latest Python developments, libraries, and best practices by reading blogs, attending meetups, and participating in online communities.
- Experiment with new technologies and techniques in a safe, isolated environment, such as a separate virtual environment or a personal project.
Conclusion
Setting up a robust and efficient Python development environment is essential for any Python developer, whether you’re a beginner or an experienced programmer. By choosing the right IDE, installing Python, setting up virtual environments, managing dependencies, and configuring version control, you can create a development workflow that is tailored to your needs and helps you be more productive.
Remember, the key to successful Python development is a combination of technical skills, best practices, and continuous learning. Keep exploring, experimenting, and refining your development environment, and you’ll be well on your way to creating amazing Python applications.